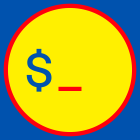 |
Livl Shell
0.1
Livl Shell is an intermediate reimplementation of the bash shell in C language.
|
Go to the documentation of this file.
7 #ifndef BUILTIN_COMMANDS_H
8 #define BUILTIN_COMMANDS_H
36 void cd(
const char *path);
60 #endif // BUILTIN_COMMANDS_H
Struct that represents a command sequence.
Definition: typedef.h:123
void echo(const Command *command)
Prints a message.
Definition: builtin_commands.c:40
Managing livl-shell aliases.
Struct that represents a command.
Definition: typedef.h:97
int execute_builtin_command(const Command *command, CommandSequence *sequence)
Executes a command.
Definition: builtin_commands.c:49
void cd(const char *path)
Changes the current directory.
Definition: builtin_commands.c:8
File containing the command sequence functions to manage the command sequence (commands and operators...
int is_builtin_command(const Command *command, const char *expected)
Checks if the command is a built-in command.
Definition: builtin_commands.c:3
int exit_shell(CommandSequence *sequence, int exit_code)
Exits the shell.
Definition: builtin_commands.c:29
void pwd()
Prints the current working directory.
Definition: builtin_commands.c:16
Header file defining constants.
File containing all the typedefs used in the shell program.